300
|
How can I change the font for entire item
excombobox1.Columns.Add("Default");
excombobox1.Items.AddItem("default font");
stdole.IFontDisp f = new stdole.StdFont();
f.Name = "Tahoma";
f.Size = 12;
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemFont(var_Items.AddItem("new font"),(f as stdole.IFontDisp));
|
299
|
How do I vertically align a cell
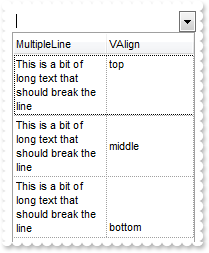
excombobox1.DrawGridLines = exontrol.EXCOMBOBOXLib.GridLinesEnum.exRowLines;
(excombobox1.Columns.Add("MultipleLine") as exontrol.EXCOMBOBOXLib.Column).set_Def(exontrol.EXCOMBOBOXLib.DefColumnEnum.exCellSingleLine,false);
excombobox1.Columns.Add("VAlign");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("This is a bit of long text that should break the line");
var_Items.set_CellCaption(h,1,"top");
var_Items.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exTop);
h = var_Items.AddItem("This is a bit of long text that should break the line");
var_Items.set_CellCaption(h,1,"middle");
var_Items.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exMiddle);
h = var_Items.AddItem("This is a bit of long text that should break the line");
var_Items.set_CellCaption(h,1,"bottom");
var_Items.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exBottom);
|
298
|
How can I change the position of an item
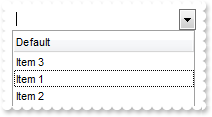
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.AddItem("Item 1");
var_Items.AddItem("Item 2");
var_Items.set_ItemPosition(var_Items.AddItem("Item 3"),0);
|
297
|
How do I find an item based on a path
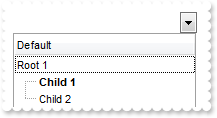
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.set_ItemData(var_Items.InsertItem(h,null,"Child 2"),1234);
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.get_FindPath("Root 1\\Child 1"),true);
|
296
|
How do I find an item
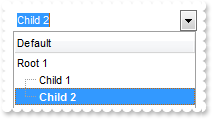
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.get_FindItem("Child 2",0,null),true);
|
295
|
How can I insert a hyperlink or an anchor element
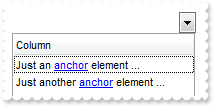
excombobox1.Columns.Add("Column");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaptionFormat(var_Items.AddItem("Just an <a1>anchor</a> element ..."),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
exontrol.EXCOMBOBOXLib.Items var_Items1 = excombobox1.Items;
var_Items1.set_CellCaptionFormat(var_Items1.AddItem("Just another <a2>anchor</a> element ..."),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
294
|
How do I find the index of the item based on its handle
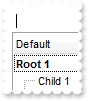
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items[var_Items.get_ItemToIndex(h)],true);
|
293
|
How do I find the handle of the item based on its index

excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items[1],true);
|
292
|
How can I find the cell being clicked in a radio group
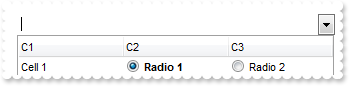
excombobox1.MarkSearchColumn = false;
excombobox1.SelBackColor = Color.FromArgb(255,255,128);
excombobox1.SelForeColor = Color.FromArgb(0,0,0);
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
excombobox1.Columns.Add("C3");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Radio 1");
var_Items.set_CellHasRadioButton(h,1,true);
var_Items.set_CellRadioGroup(h,1,1234);
var_Items.set_CellCaption(h,2,"Radio 2");
var_Items.set_CellHasRadioButton(h,2,true);
var_Items.set_CellRadioGroup(h,2,1234);
var_Items.set_CellState(h,1,1);
var_Items.set_CellBold(null,var_Items.get_CellChecked(1234),true);
|
291
|
Can I add a +/- ( expand / collapse ) buttons to each item, so I can load the child items later
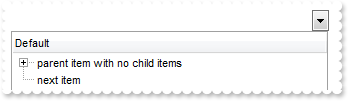
excombobox1.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot;
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemHasChildren(var_Items.AddItem("parent item with no child items"),true);
var_Items.AddItem("next item");
|
290
|
Can I let the user to resize at runtime the specified item
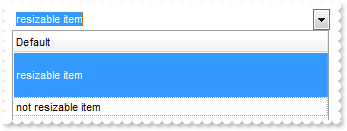
excombobox1.ScrollBySingleLine = true;
excombobox1.DrawGridLines = exontrol.EXCOMBOBOXLib.GridLinesEnum.exRowLines;
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemAllowSizing(var_Items.AddItem("resizable item"),true);
var_Items.AddItem("not resizable item");
|
289
|
How can I change the size ( width, height ) of the picture
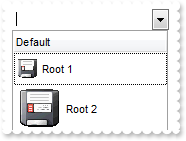
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.set_CellPicture(h,0,(excombobox1.ExecuteTemplate("loadpicture(`c:\\exontrol\\images\\zipdisk.gif`)") as stdole.IPictureDisp));
var_Items.set_CellPictureWidth(h,0,24);
var_Items.set_CellPictureHeight(h,0,24);
var_Items.set_ItemHeight(h,32);
h = var_Items.AddItem("Root 2");
var_Items.set_CellPicture(h,0,(excombobox1.ExecuteTemplate("loadpicture(`c:\\exontrol\\images\\zipdisk.gif`)") as stdole.IPictureDisp));
var_Items.set_ItemHeight(h,48);
|
288
|
How do I unselect an item
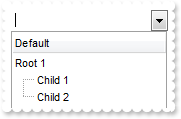
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_SelectItem(h,false);
|
287
|
How do I find the selected item
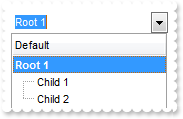
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_SelectItem(h,true);
var_Items.set_ItemBold(var_Items.get_SelectedItem(0),true);
|
286
|
How do I select an item
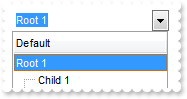
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_SelectItem(h,true);
|
285
|
Can I display a button with some picture or icon inside
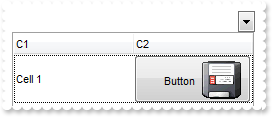
excombobox1.set_HTMLPicture("p1","c:\\exontrol\\images\\zipdisk.gif");
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1," Button <img>p1</img> ");
var_Items.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
var_Items.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment);
var_Items.set_CellHasButton(h,1,true);
var_Items.set_ItemHeight(h,48);
|
284
|
Can I display a button with some picture or icon inside
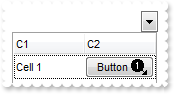
excombobox1.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1," Button <img>1</img> ");
var_Items.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
var_Items.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment);
var_Items.set_CellHasButton(h,1,true);
|
283
|
Can I display a button with some icon inside
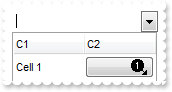
excombobox1.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1," <img>1</img> ");
var_Items.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
var_Items.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment);
var_Items.set_CellHasButton(h,1,true);
|
282
|
How can I assign multiple icon/picture to a cell
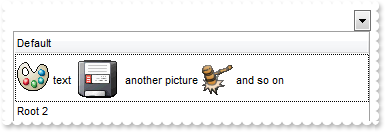
excombobox1.set_HTMLPicture("p1","c:\\exontrol\\images\\zipdisk.gif");
excombobox1.set_HTMLPicture("p2","c:\\exontrol\\images\\auction.gif");
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("text <img>p1</img> another picture <img>p2</img> and so on");
var_Items.set_CellCaptionFormat(h,0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
var_Items.set_CellPicture(h,0,(excombobox1.ExecuteTemplate("loadpicture(`c:\\exontrol\\images\\colorize.gif`)") as stdole.IPictureDisp));
var_Items.set_ItemHeight(h,48);
var_Items.AddItem("Root 2");
|
281
|
How can I assign an icon/picture to a cell
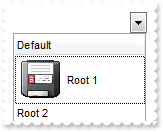
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.set_CellPicture(h,0,(excombobox1.ExecuteTemplate("loadpicture(`c:\\exontrol\\images\\zipdisk.gif`)") as stdole.IPictureDisp));
var_Items.set_ItemHeight(h,48);
var_Items.AddItem("Root 2");
|
280
|
How can I assign multiple icons/pictures to a cell
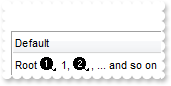
excombobox1.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root <img>1</img> 1, <img>2</img>, ... and so on ");
var_Items.set_CellCaptionFormat(h,0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
279
|
How can I assign multiple icons/pictures to a cell
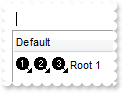
excombobox1.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.set_CellImages(h,0,"1,2,3");
|
278
|
How can I assign an icon/picture to a cell
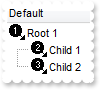
excombobox1.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.set_CellImage(h,0,1);
var_Items.set_CellImage(var_Items.InsertItem(h,null,"Child 1"),0,2);
var_Items.set_CellImage(var_Items.InsertItem(h,null,"Child 2"),0,3);
var_Items.set_ExpandItem(h,true);
|
277
|
How can I get the handle of an item based on the handle of the cell
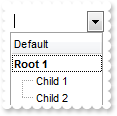
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.get_CellItem(var_Items.get_ItemCell(h,0)),true);
|
276
|
How can I display a button inside the item or cell
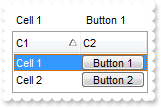
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1," Button 1 ");
var_Items.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment);
var_Items.set_CellHasButton(h,1,true);
h = var_Items.AddItem("Cell 2");
var_Items.set_CellCaption(h,1," Button 2 ");
var_Items.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.CenterAlignment);
var_Items.set_CellHasButton(h,1,true);
|
275
|
How can I change the state of a radio button
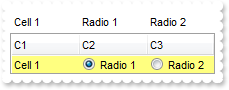
excombobox1.MarkSearchColumn = false;
excombobox1.SelBackColor = Color.FromArgb(255,255,128);
excombobox1.SelForeColor = Color.FromArgb(0,0,0);
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
excombobox1.Columns.Add("C3");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Radio 1");
var_Items.set_CellHasRadioButton(h,1,true);
var_Items.set_CellRadioGroup(h,1,1234);
var_Items.set_CellCaption(h,2,"Radio 2");
var_Items.set_CellHasRadioButton(h,2,true);
var_Items.set_CellRadioGroup(h,2,1234);
var_Items.set_CellState(h,1,1);
|
274
|
How can I assign a radio button to a cell
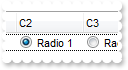
excombobox1.MarkSearchColumn = false;
excombobox1.SelBackColor = Color.FromArgb(255,255,128);
excombobox1.SelForeColor = Color.FromArgb(0,0,0);
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
excombobox1.Columns.Add("C3");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Radio 1");
var_Items.set_CellHasRadioButton(h,1,true);
var_Items.set_CellRadioGroup(h,1,1234);
var_Items.set_CellCaption(h,2,"Radio 2");
var_Items.set_CellHasRadioButton(h,2,true);
var_Items.set_CellRadioGroup(h,2,1234);
var_Items.set_CellState(h,1,1);
|
273
|
How can I change the state of a checkbox
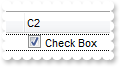
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Check Box");
var_Items.set_CellHasCheckBox(h,1,true);
var_Items.set_CellState(h,1,1);
|
272
|
How can I assign a checkbox to a cell
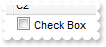
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Check Box");
var_Items.set_CellHasCheckBox(h,1,true);
|
271
|
How can I display an item or a cell on multiple lines
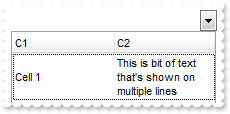
excombobox1.ScrollBySingleLine = true;
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"This is bit of text that's shown on multiple lines");
var_Items.set_CellSingleLine(h,1,exontrol.EXCOMBOBOXLib.CellSingleLineEnum.exCaptionWordWrap);
|
270
|
How can I assign a tooltip to a cell
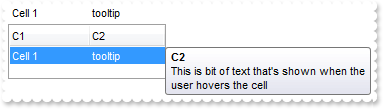
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"tooltip");
var_Items.set_CellToolTip(h,1,"This is bit of text that's shown when the user hovers the cell");
|
269
|
How can I associate an extra data to a cell
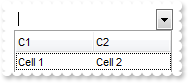
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellData(h,1,"your extra data");
|
268
|
How do I enable or disable a cell
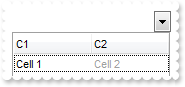
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellEnabled(h,1,false);
|
267
|
How do I change the cell's foreground color
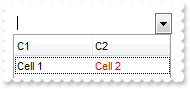
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellForeColor(h,1,Color.FromArgb(255,0,0));
|
266
|
How do I change the visual effect for the cell, using your EBN files
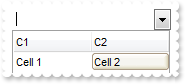
excombobox1.VisualAppearance.Add(1,"c:\\exontrol\\images\\normal.ebn");
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellBackColor32(h,1,0x1000000);
|
265
|
How do I change the cell's background color
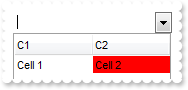
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellBackColor(h,1,Color.FromArgb(255,0,0));
|
264
|
How do I change the caption or value for a particular cell
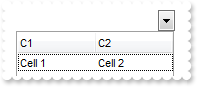
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaption(var_Items.AddItem("Cell 1"),1,"Cell 2");
|
263
|
How do I get the handle of the cell
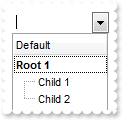
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_CellBold(null,var_Items.get_ItemCell(h,0),true);
|
262
|
How do I retrieve the focused item
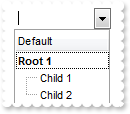
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.FocusItem,true);
|
261
|
How do I get the number or count of child items
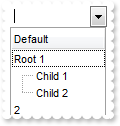
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.AddItem(var_Items.get_ChildCount(h));
|
260
|
How do I enumerate the visible items
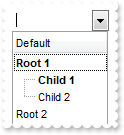
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
h = var_Items.AddItem("Root 2");
var_Items.set_ItemBold(var_Items.FirstVisibleItem,true);
var_Items.set_ItemBold(var_Items.get_NextVisibleItem(var_Items.FirstVisibleItem),true);
|
259
|
How do I enumerate the siblings items
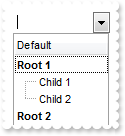
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
h = var_Items.AddItem("Root 2");
var_Items.set_ItemBold(var_Items.get_NextSiblingItem(var_Items.FirstVisibleItem),true);
var_Items.set_ItemBold(var_Items.get_PrevSiblingItem(var_Items.get_NextSiblingItem(var_Items.FirstVisibleItem)),true);
|
258
|
How do I get the parent item
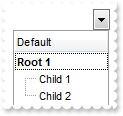
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.get_ItemParent(var_Items.get_ItemChild(h)),true);
|
257
|
How do I get the first child item
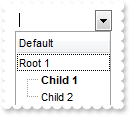
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.set_ItemBold(var_Items.get_ItemChild(h),true);
|
256
|
How do I enumerate the root items
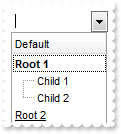
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
h = var_Items.AddItem("Root 2");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ItemBold(var_Items.get_RootItem(0),true);
var_Items.set_ItemUnderline(var_Items.get_RootItem(1),true);
|
255
|
I have a hierarchy, how can I count the number of root items
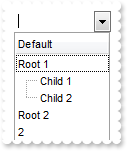
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root 1");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
h = var_Items.AddItem("Root 2");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.AddItem(var_Items.RootCount);
|
254
|
How can I make an item unselectable, or not selectable
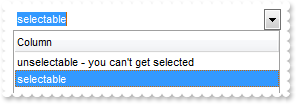
excombobox1.Columns.Add("Column");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("unselectable - you can't get selected");
var_Items.set_SelectableItem(h,false);
var_Items.AddItem("selectable");
|
253
|
How can I hide or show an item
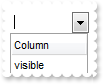
excombobox1.Columns.Add("Column");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("hidden");
var_Items.set_ItemHeight(h,0);
var_Items.set_SelectableItem(h,false);
var_Items.AddItem("visible");
|
252
|
How can I change the height for all items
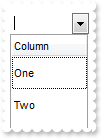
excombobox1.DefaultItemHeight = 32;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem("One");
excombobox1.Items.AddItem("Two");
|
251
|
How do I change the height of an item
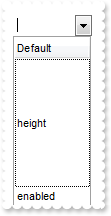
excombobox1.ScrollBySingleLine = true;
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemHeight(var_Items.AddItem("height"),128);
excombobox1.Items.AddItem("enabled");
|
250
|
How do I disable or enable an item
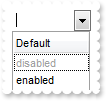
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_EnableItem(var_Items.AddItem("disabled"),false);
excombobox1.Items.AddItem("enabled");
|
249
|
How do I display as strikeout a cell
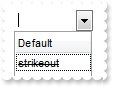
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellStrikeOut(var_Items.AddItem("strikeout"),0,true);
|
248
|
How do I display as strikeout a cell or an item
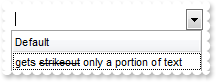
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaptionFormat(var_Items.AddItem("gets <s>strikeout</s> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
247
|
How do I display as strikeout an item
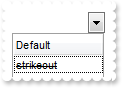
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemStrikeOut(var_Items.AddItem("strikeout"),true);
|
246
|
How do I underline a cell
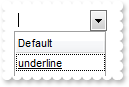
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellUnderline(var_Items.AddItem("underline"),0,true);
|
245
|
How do I underline a cell or an item
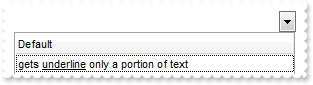
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaptionFormat(var_Items.AddItem("gets <u>underline</u> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
244
|
How do I underline an item
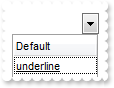
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemUnderline(var_Items.AddItem("underline"),true);
|
243
|
How do I display as italic a cell
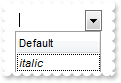
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellItalic(var_Items.AddItem("italic"),0,true);
|
242
|
How do I display as italic a cell or an item
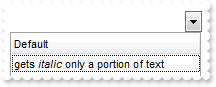
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaptionFormat(var_Items.AddItem("gets <i>italic</i> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
241
|
How do I display as italic an item

excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemItalic(var_Items.AddItem("italic"),true);
|
240
|
How do I bold a cell

excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellBold(var_Items.AddItem("bold"),0,true);
|
239
|
How do I bold a cell or an item
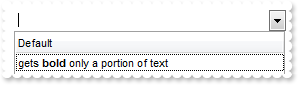
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaptionFormat(var_Items.AddItem("gets <b>bold</b> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML);
|
238
|
How do I bold an item
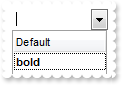
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemBold(var_Items.AddItem("bold"),true);
|
237
|
How do I change the foreground color for the item
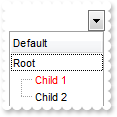
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
int hC = var_Items.InsertItem(h,null,"Child 1");
var_Items.set_ItemForeColor(hC,Color.FromArgb(255,0,0));
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
|
236
|
How do I change the visual appearance for the item, using your EBN technology
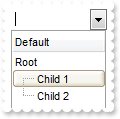
excombobox1.VisualAppearance.Add(1,"c:\\exontrol\\images\\normal.ebn");
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
int hC = var_Items.InsertItem(h,null,"Child 1");
var_Items.set_ItemBackColor32(hC,0x1000000);
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
|
235
|
How do I change the background color for the item
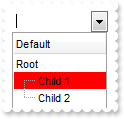
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
int hC = var_Items.InsertItem(h,null,"Child 1");
var_Items.set_ItemBackColor(hC,Color.FromArgb(255,0,0));
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
|
234
|
How do I expand or collapse an item
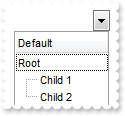
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
|
233
|
How do I associate an extra data to an item
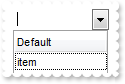
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_ItemData(var_Items.AddItem("item"),"your extra data");
|
232
|
How do I get the number or count of items
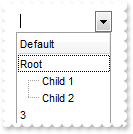
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
exontrol.EXCOMBOBOXLib.Items var_Items1 = excombobox1.Items;
var_Items1.AddItem(var_Items1.ItemCount);
|
231
|
How can I change at runtime the parent of the item
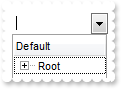
excombobox1.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot;
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int hP = var_Items.AddItem("Root");
int hC = var_Items.AddItem("Child");
var_Items.SetParent(hC,hP);
|
230
|
How can I sort the items
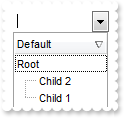
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
excombobox1.Columns["Default"].SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortDescending;
|
229
|
How do I sort the child items
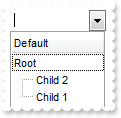
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Root");
var_Items.InsertItem(h,null,"Child 1");
var_Items.InsertItem(h,null,"Child 2");
var_Items.set_ExpandItem(h,true);
var_Items.SortChildren(h,0,false);
|
228
|
How can I remove or delete all items
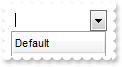
excombobox1.Columns.Add("Default");
excombobox1.Items.AddItem("removed item");
excombobox1.Items.RemoveAllItems();
|
227
|
How can I remove or delete an item
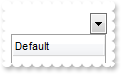
excombobox1.Columns.Add("Default");
int h = excombobox1.Items.AddItem("removed item");
excombobox1.Items.RemoveItem(h);
|
226
|
How can I add or insert child items
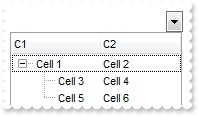
excombobox1.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot;
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
int h = var_Items.AddItem("Cell 1");
var_Items.set_CellCaption(h,1,"Cell 2");
var_Items.set_CellCaption(var_Items.InsertItem(h,null,"Cell 3"),1,"Cell 4");
var_Items.set_CellCaption(var_Items.InsertItem(h,null,"Cell 5"),1,"Cell 6");
var_Items.set_ExpandItem(h,true);
|
225
|
How can I add or insert a child item
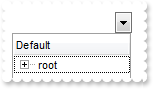
excombobox1.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot;
excombobox1.Columns.Add("Default");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.InsertItem(var_Items.AddItem("root"),null,"child");
|
224
|
How can I add or insert an item
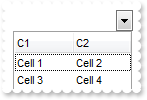
excombobox1.Columns.Add("C1");
excombobox1.Columns.Add("C2");
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaption(var_Items.AddItem("Cell 1"),1,"Cell 2");
int h = var_Items.AddItem("Cell 3");
var_Items.set_CellCaption(h,1,"Cell 4");
|
223
|
How can I add or insert an item
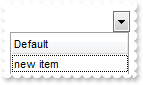
excombobox1.Columns.Add("Default");
excombobox1.Items.AddItem("new item");
|
222
|
How can I get the columns as they are shown in the control's sortbar
Object var_Object = (excombobox1.Columns.get_ItemBySortPosition(0) as Object);
|
221
|
How can I access the properties of a column
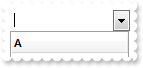
excombobox1.Columns.Add("A");
excombobox1.Columns["A"].HeaderBold = true;
|
220
|
How can I remove all the columns
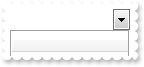
excombobox1.Columns.Clear();
|
219
|
How can I remove a column
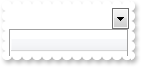
excombobox1.Columns.Remove("A");
|
218
|
How can I get the number or the count of columns
int var_Count = excombobox1.Columns.Count;
|
217
|
How can I change the font for all cells in the entire column
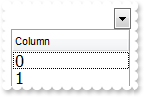
stdole.IFontDisp f = new stdole.StdFont();
f.Name = "Tahoma";
f.Size = 12;
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.Font = (f as stdole.IFontDisp);
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
216
|
How can I change the background color for all cells in the column
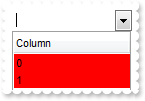
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.BackColor = Color.FromArgb(255,0,0);
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
215
|
How can I change the foreground color for all cells in the column
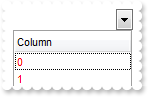
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.ForeColor = Color.FromArgb(255,0,0);
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
214
|
How can I show as strikeout all cells in the column
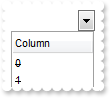
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.StrikeOut = true;
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
213
|
How can I underline all cells in the column
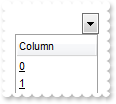
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.Underline = true;
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
212
|
How can I show in italic all data in the column
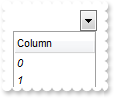
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.Italic = true;
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
211
|
How can I bold the entire column
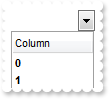
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("1",null);
var_ConditionalFormat.Bold = true;
var_ConditionalFormat.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns;
excombobox1.Columns.Add("Column");
excombobox1.Items.AddItem(0);
excombobox1.Items.AddItem(1);
|
210
|
How can I display a computed column and highlight some values that are negative or less than a value
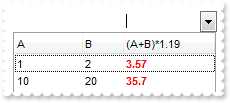
excombobox1.Columns.Add("A");
excombobox1.Columns.Add("B");
(excombobox1.Columns.Add("(A+B)*1.19") as exontrol.EXCOMBOBOXLib.Column).ComputedField = "(%0 + %1) * 1.19";
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaption(var_Items.AddItem(1),1,2);
exontrol.EXCOMBOBOXLib.Items var_Items1 = excombobox1.Items;
var_Items1.set_CellCaption(var_Items1.AddItem(10),1,20);
exontrol.EXCOMBOBOXLib.ConditionalFormat var_ConditionalFormat = excombobox1.ConditionalFormats.Add("%2 > 10",null);
var_ConditionalFormat.Bold = true;
var_ConditionalFormat.ForeColor = Color.FromArgb(255,0,0);
var_ConditionalFormat.ApplyTo = (exontrol.EXCOMBOBOXLib.FormatApplyToEnum)0x2;
|
209
|
Can I display a computed column so it displays the VAT, or SUM
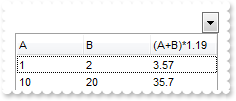
excombobox1.Columns.Add("A");
excombobox1.Columns.Add("B");
(excombobox1.Columns.Add("(A+B)*1.19") as exontrol.EXCOMBOBOXLib.Column).ComputedField = "(%0 + %1) * 1.19";
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaption(var_Items.AddItem(1),1,2);
exontrol.EXCOMBOBOXLib.Items var_Items1 = excombobox1.Items;
var_Items1.set_CellCaption(var_Items1.AddItem(10),1,20);
|
208
|
How can I show a column that adds values in the cells
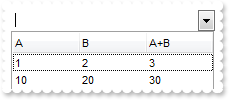
excombobox1.Columns.Add("A");
excombobox1.Columns.Add("B");
(excombobox1.Columns.Add("A+B") as exontrol.EXCOMBOBOXLib.Column).ComputedField = "%0 + %1";
exontrol.EXCOMBOBOXLib.Items var_Items = excombobox1.Items;
var_Items.set_CellCaption(var_Items.AddItem(1),1,2);
exontrol.EXCOMBOBOXLib.Items var_Items1 = excombobox1.Items;
var_Items1.set_CellCaption(var_Items1.AddItem(10),1,20);
|
207
|
Is there any function to filter the control's data as I type, so the items being displayed include the typed characters
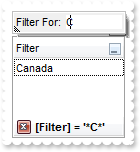
exontrol.EXCOMBOBOXLib.Column var_Column = (excombobox1.Columns.Add("Filter") as exontrol.EXCOMBOBOXLib.Column);
var_Column.FilterOnType = true;
var_Column.DisplayFilterButton = true;
var_Column.AutoSearch = exontrol.EXCOMBOBOXLib.AutoSearchEnum.exContains;
excombobox1.Items.AddItem("Canada");
excombobox1.Items.AddItem("USA");
|
206
|
Is there any function to filter the control's data as I type, something like filter on type
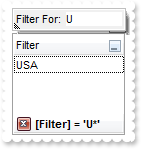
exontrol.EXCOMBOBOXLib.Column var_Column = (excombobox1.Columns.Add("Filter") as exontrol.EXCOMBOBOXLib.Column);
var_Column.FilterOnType = true;
var_Column.DisplayFilterButton = true;
excombobox1.Items.AddItem("Canada");
excombobox1.Items.AddItem("USA");
|
205
|
How can I programmatically filter a column
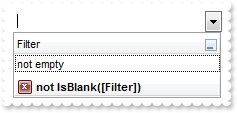
exontrol.EXCOMBOBOXLib.Column var_Column = (excombobox1.Columns.Add("Filter") as exontrol.EXCOMBOBOXLib.Column);
var_Column.DisplayFilterButton = true;
var_Column.FilterType = exontrol.EXCOMBOBOXLib.FilterTypeEnum.exNonBlanks;
excombobox1.Items.AddItem(null);
excombobox1.Items.AddItem("not empty");
excombobox1.ApplyFilter();
|
204
|
How can I show or display the control's filter
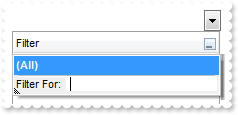
(excombobox1.Columns.Add("Filter") as exontrol.EXCOMBOBOXLib.Column).DisplayFilterButton = true;
|
203
|
How can I customize the items being displayed in the drop down filter window
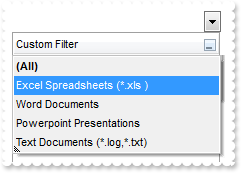
exontrol.EXCOMBOBOXLib.Column var_Column = (excombobox1.Columns.Add("Custom Filter") as exontrol.EXCOMBOBOXLib.Column);
var_Column.DisplayFilterButton = true;
var_Column.DisplayFilterPattern = false;
var_Column.CustomFilter = "Excel Spreadsheets (*.xls )||*.xls|||Word Documents||*.doc|||Powerpoint Presentations||*.pps|||Text Documents (*.log,*.txt)||*." +
"txt|*.log";
var_Column.FilterType = exontrol.EXCOMBOBOXLib.FilterTypeEnum.exPattern;
var_Column.Filter = "*.xls";
excombobox1.Items.AddItem("excel.xls");
excombobox1.Items.AddItem("word.doc");
excombobox1.Items.AddItem("pp.pps");
excombobox1.Items.AddItem("text.txt");
excombobox1.ApplyFilter();
|
202
|
How can I change the order or the position of the columns in the sort bar
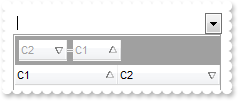
excombobox1.SortBarVisible = true;
excombobox1.SortBarColumnWidth = 48;
(excombobox1.Columns.Add("C1") as exontrol.EXCOMBOBOXLib.Column).SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortAscending;
(excombobox1.Columns.Add("C2") as exontrol.EXCOMBOBOXLib.Column).SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortDescending;
excombobox1.Columns["C2"].SortPosition = 0;
|
201
|
How do I arrange my columns on multiple levels
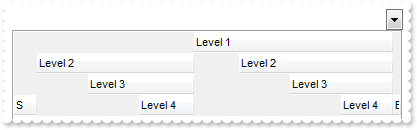
(excombobox1.Columns.Add("S") as exontrol.EXCOMBOBOXLib.Column).Width = 32;
(excombobox1.Columns.Add("Level 2") as exontrol.EXCOMBOBOXLib.Column).LevelKey = 1;
(excombobox1.Columns.Add("Level 3") as exontrol.EXCOMBOBOXLib.Column).LevelKey = 1;
(excombobox1.Columns.Add("Level 4") as exontrol.EXCOMBOBOXLib.Column).LevelKey = 1;
(excombobox1.Columns.Add("Level 1") as exontrol.EXCOMBOBOXLib.Column).LevelKey = "2";
(excombobox1.Columns.Add("Level 2") as exontrol.EXCOMBOBOXLib.Column).LevelKey = "2";
(excombobox1.Columns.Add("Level 3") as exontrol.EXCOMBOBOXLib.Column).LevelKey = "2";
(excombobox1.Columns.Add("Level 4") as exontrol.EXCOMBOBOXLib.Column).LevelKey = "2";
(excombobox1.Columns.Add("E") as exontrol.EXCOMBOBOXLib.Column).Width = 32;
|